黄金行情分析软件(Java)
作者
黄金价格数据API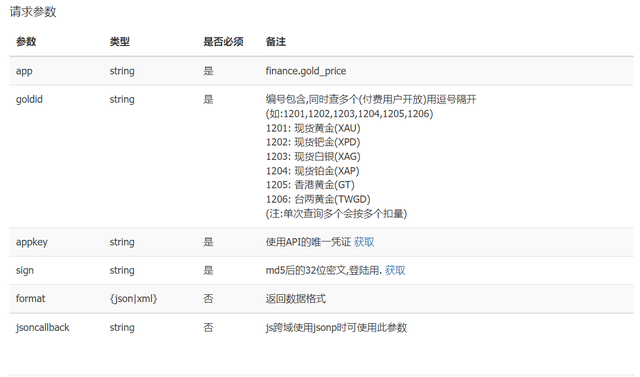
API提供方:https://www.nowapi.com/
国际金价实时(盎司):https://www.nowapi.com/api/finance.gsgold
国际金价实时(盎司)
作用:查询上海黄金交易所黄金价格、国际公制金价、国际贵金属期货价、工行纸黄金等。
测试示例: http://api.k780.com/?app=finance.gold_price&goldid=1201&appkey=10003&sign=b59bc3ef6191eb9f747dd4e83c99f2a4&format=json (示例中sign会不定期调整)
调用参数:
返回格式:
{ "result": { "dtList": { "1201": { "sell_price": "1716.73", "high_price": "1719.2", "goldid": "1201", "uptime": "2022-07-22 13:58:01", "varietynm": "现货黄金", "variety": "XAU", "change_margin": "-0.14%", "yesy_price": "1718.65", "buy_price": "1716.3", "change_price": "-2.35", "open_price": "1717.84", "low_price": "1712.77", "last_price": "1716.3" } }, "dtQuery": "1201", "dtCount": "1" }, "success": "1"}调用代码案例pom.xml
<dependencies> <dependency> <groupId>cn.hutool</groupId> <artifactId>hutool-all</artifactId> <version>5.5.9</version> </dependency> <dependency> <groupId>org.apache.httpcomponents.client5</groupId> <artifactId>httpclient5</artifactId> <version>5.1</version> </dependency> <dependency> <groupId>org.apache.httpcomponents.client5</groupId> <artifactId>httpclient5-fluent</artifactId> <version>5.0.3</version> </dependency></dependencies>调用方式1:
import org.apache.commons.codec.Charsets;import org.apache.hc.client5.http.classic.methods.HttpPost;import org.apache.hc.client5.http.entity.UrlEncodedFormEntity;import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;import org.apache.hc.client5.http.impl.classic.HttpClients;import org.apache.hc.core5.http.HttpEntity;import org.apache.hc.core5.http.NameValuePair;import org.apache.hc.core5.http.io.entity.EntityUtils;import org.apache.hc.core5.http.message.BasicNameValuePair;import java.util.ArrayList;import java.util.List;public class HttpClientClassicApp { public static void main(String[] args) throws Exception { CloseableHttpClient httpClient = HttpClients.createDefault(); HttpPost httpPost = new HttpPost("http://api.k780.com/"); List<NameValuePair> nvps = new ArrayList<>(); nvps.add(new BasicNameValuePair("app", "finance.gold_price")); nvps.add(new BasicNameValuePair("goldid", "1201")); nvps.add(new BasicNameValuePair("appkey", "10003")); nvps.add(new BasicNameValuePair("sign", "b59bc3ef6191eb9f747dd4e83c99f2a4")); nvps.add(new BasicNameValuePair("format", "json")); httpPost.setEntity(new UrlEncodedFormEntity(nvps, Charsets.UTF_8)); CloseableHttpResponse response = httpClient.execute(httpPost); System.out.println(response.getCode() + " " + response.getReasonPhrase()); HttpEntity entity = response.getEntity(); String content = EntityUtils.toString(entity); System.out.println(content); EntityUtils.consume(entity); }}调用方式2:
import cn.hutool.json.JSONObject;import cn.hutool.json.JSONUtil;import org.apache.hc.client5.http.async.methods.*;import org.apache.hc.client5.http.config.RequestConfig;import org.apache.hc.client5.http.impl.async.CloseableHttpAsyncClient;import org.apache.hc.client5.http.impl.async.HttpAsyncClients;import org.apache.hc.client5.http.impl.nio.PoolingAsyncClientConnectionManager;import org.apache.hc.client5.http.impl.nio.PoolingAsyncClientConnectionManagerBuilder;import org.apache.hc.core5.concurrent.FutureCallback;import org.apache.hc.core5.http.Method;import org.apache.hc.core5.http.message.StatusLine;import org.apache.hc.core5.pool.PoolConcurrencyPolicy;import org.apache.hc.core5.reactor.IOReactorConfig;import org.apache.hc.core5.util.TimeValue;import org.apache.hc.core5.util.Timeout;import java.util.concurrent.Future;import java.util.concurrent.TimeUnit;public class HttpClient5App { public static void main(String[] args) { // 异步应用配置 IOReactorConfig ioReactorConfig = IOReactorConfig.custom() .setSoTimeout(Timeout.ofMilliseconds(250)) // 超时时间 .setSelectInterval(TimeValue.ofMilliseconds(50)) // 1.2 .build(); // setSoTimeout()====>连接上一个url,获取response的返回等待时间 // setSelectInterval()====>NIO中设置select的间隔 // 客户端连接配置 PoolingAsyncClientConnectionManager build = PoolingAsyncClientConnectionManagerBuilder.create() .setPoolConcurrencyPolicy(PoolConcurrencyPolicy.LAX) // 2.1 .setMaxConnPerRoute(6).build(); // 2.2 // poolConcurrencyPolicy() ====> STRICT模式通过加锁的方式对,LAX通过cas的方式宽松计数 // 设置STRICT,会使用StrictConnPool实现类;设置LAX ,会使用LaxConnPool实现类 // maxConnPerRoute ====> 每个route最多能有多少个connection // 创建客户端 CloseableHttpAsyncClient client = HttpAsyncClients.custom() .setIOReactorConfig(ioReactorConfig) .setConnectionManager(build) .disableAutomaticRetries() .build(); // automaticRetriesDisabled===>关闭自动重试 client.start(); String uri = "http://api.k780.com/"; SimpleHttpRequest httpRequest = SimpleHttpRequest.create(Method.GET.name(), uri); final SimpleHttpRequest request = SimpleRequestBuilder.copy(httpRequest) .addParameter("app", "finance.gold_price") .addParameter("goldid", "1201") .addParameter("appkey", "10003") .addParameter("sign", "b59bc3ef6191eb9f747dd4e83c99f2a4") .addParameter("format", "json") .build(); request.setHeader("Host", "api.k780.com"); RequestConfig config = RequestConfig.copy(RequestConfig.DEFAULT) .setConnectTimeout(150, TimeUnit.MILLISECONDS) .setConnectionRequestTimeout(200, TimeUnit.MILLISECONDS) .setResponseTimeout(100, TimeUnit.MILLISECONDS).build(); request.setConfig(config); long start = System.currentTimeMillis(); final Future<SimpleHttpResponse> future = client.execute( SimpleRequestProducer.create(request), SimpleResponseConsumer.create(), new FutureCallback<SimpleHttpResponse>() { @Override public void completed(final SimpleHttpResponse response) { System.out.println(request + "->" + new StatusLine(response)); System.out.println(response.getBody()); } @Override public void failed(final Exception ex) { System.out.println(request + "->" + ex); } @Override public void cancelled() { System.out.println(request + " cancelled"); } }); // 时间计算 try { SimpleHttpResponse simpleHttpResponse = future.get(); JSONObject jsonObject = JSONUtil.parseObj(simpleHttpResponse.getBodyText()); System.out.println("body=" + jsonObject); long end = System.currentTimeMillis(); System.out.println("时间差:" + (end - start)); } catch (Exception e) { long end = System.currentTimeMillis(); System.out.println("时间差:" + (end - start)); System.out.println(e); } // 线程等待 try { Thread.sleep(10000); } catch (InterruptedException e) { e.printStackTrace(); } }}目录